Making API Requests
Now that you've gone over the authentication process and the Connect flow, you can explore making API calls to gain access to the financial data of a connected business. As an example, we'll look at making API calls to:
- Checking data synchronization status.
- Retrieving synchronized data.
- Pushing data.
API Authentication
Accounting Data as a Service™ expects an authentication token to be included in all API requests to the server. Refer to the Authentication section in API Reference for more information.
Checking Data Synchronization Status
-
You can check the last time each data type was synchronized using the GET /data/syncStatus endpoint.
-
The status for the last time each data type was synced successfully is indicated in:
lastSuccessfulSync
.
{
"connectionId": "CON-94652dff-06ab-4b97-bd1b-f91adf7048c3",
"dataTypes":
[
{
"dataType": "BalanceSheet",
"reports":
[
{
"startDate": "2021-03-01T00:00:00Z",
"endDate": "2021-03-31T00:00:00Z",
"lastSuccessfulSync": "2021-04-01T00:31:00Z",
"reportFrequency": "month",
"currentStatus": "success",
"latestReportId": "6070149d1d685e6cf0583e36",
"latestSuccessfulReportId": "601077f5914dac670966f660"
}
]
}
]
}
Retrieving Synchronized Data
Anytime you want to access your business customer financial data, you will need to use the businessName
and serviceName
associated with that business connection to make a request to our API.
As an example, we'll look at the API call to the GET /balanceSheets endpoint to retrieve the year-end 2020 balance sheet for the business connected in the previous step.
You can provide any additional query string parameters per the Balance Sheet data model.
Common Query Parameters
See pagination, sorting and filtering for more details.
curl --request GET \
--url 'https://api.railz.ai/balanceSheets?businessName=Test%20Business&reportFrequency=year&serviceName=quickbooks&startDate=2020-01-01&endDate=2020-12-31' \
--header 'Accept: application/json' \
--header 'Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJjdXN0b21lcklkIjo1LCJzYW5kYm94IjpmYWxzZSwibmFtZSI6ImFjY2VzcyIsImJldGEiOnRydWUsImlhdCI6MTYzMzc5MzU.'
import requests
url = "https://api.railz.ai/balanceSheets?businessName=Railz%20Test%20Business&reportFrequency=year&serviceName=quickbooks&startDate=2020-01-01&endDate=2020-12-31"
headers = {
"Accept": "application/json",
"Authorization": "Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJjdXN0b21lcklkIjo1LCJzYW5kYm94IjpmYWxzZSwibmFtZSI6ImFjY2VzcyIsImJldGEiOnRydWUsImlhdCI6MTYzMzc5MzU."
}
response = requests.request("GET", url, headers=headers)
print(response.text)
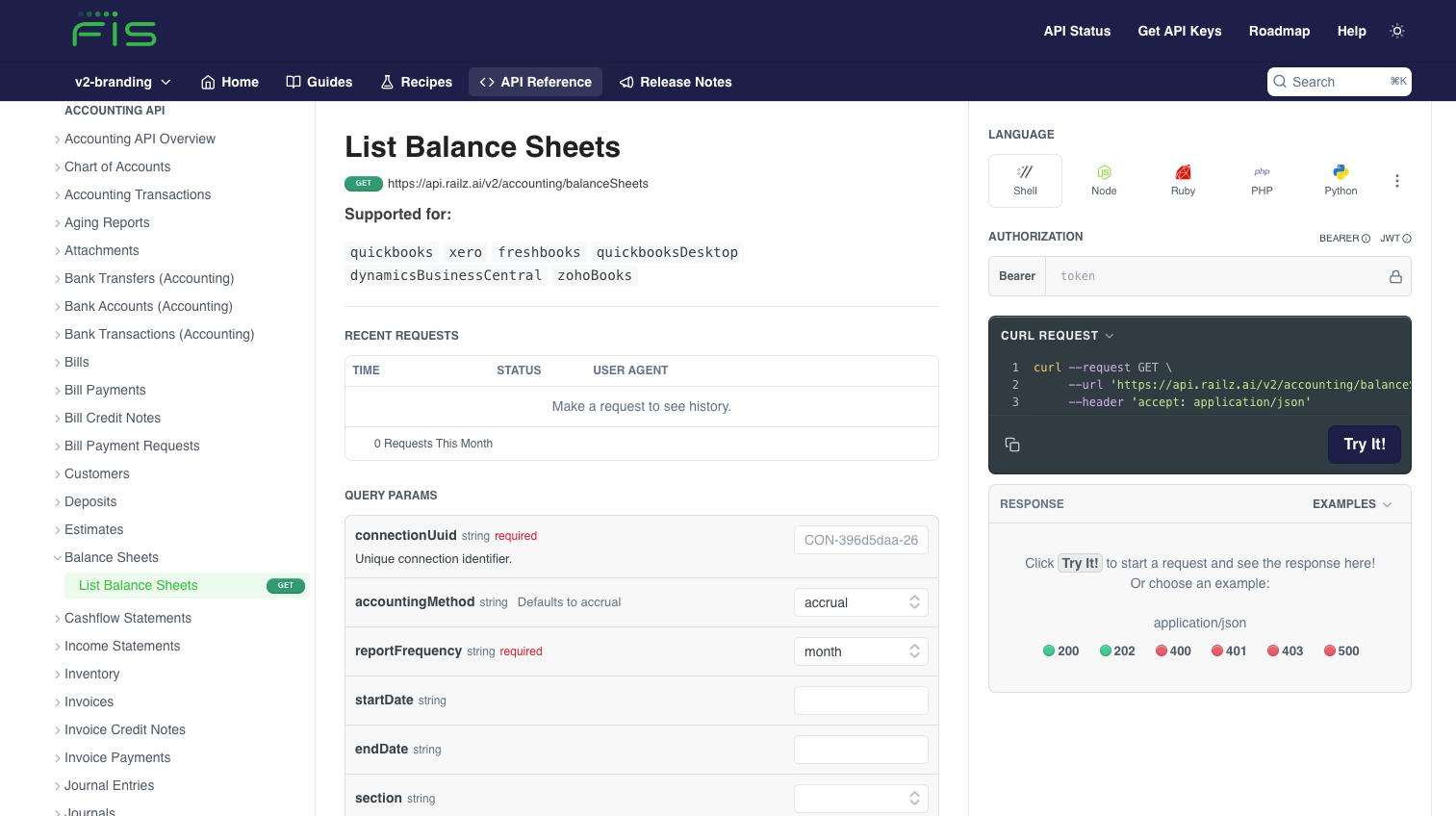
Sample request through the Accounting Data as a Service™ API reference in docs. Click to Expand.
{
"count": 2,
"reports": [
{
"meta": {
"reportId": "6000000aa6411a1f166e7543",
"startDate": "2020-01-01T00:00:00",
"endDate": "2020-12-31T00:00:00",
"serviceName": "quickbooks",
"businessName": "Test Business",
"reportFrequency": "year",
"currency": "CAD",
"createdAt": "2021-01-16T21:14:39",
"updatedAt": "2021-01-16T21:14:39"
},
"data": [
{
"section": "Assets",
"subSection": "Current Assets",
"group": "Other Current Assets",
"subGroup": "Bank Accounts",
"account": "Business Bank Account",
"value": 1612270.67
},
{
"section": "Assets",
"subSection": "Current Assets",
"group": "Other Current Assets",
"subGroup": "AR",
"account": "Accounts Receivable (A/R)",
"value": 10324.27
},
{
"section": "Assets",
"subSection": "Current Assets",
"group": "Other Current Assets",
"account": "Uncategorized Asset",
"value": -1394.37
},
{
"section": "Assets",
"subSection": "Current Assets",
"group": "Other Assets",
"subGroup": "Fixed Assets",
"account": "Accumulated Depreciation",
"value": -1500
},
{
"section": "Assets",
"subSection": "Current Assets",
"group": "Other Assets",
"subGroup": "Fixed Assets",
"account": "Furniture and Fixtures",
"value": 10000
},
{
"section": "Liabilities",
"subSection": "Current Liabilities",
"group": "Other Current Liabilities",
"subGroup": "AP",
"account": "Accounts payable (A/P)",
"value": 8078.89
},
{
"section": "Liabilities",
"subSection": "Current Liabilities",
"group": "Other Current Liabilities",
"account": "Bank Revaluations",
"value": -110
},
{
"section": "Liabilities",
"subSection": "Current Liabilities",
"group": "Other Current Liabilities",
"account": "GST/HST Payable",
"value": 5498.18
},
{
"section": "Liabilities",
"subSection": "Current Liabilities",
"group": "Other Current Liabilities",
"account": "Unrealised Currency Gains",
"value": -1091.22
},
{
"section": "Liabilities",
"subSection": "Non-Current Liabilities",
"group": "Long Term Liabilities",
"account": "Shareholder Notes Payable",
"value": 510000
},
{
"section": "Liabilities",
"subSection": "Non-Current Liabilities",
"group": "Long Term Liabilities",
"account": "Small Business Loan - BoA",
"value": 70000
},
{
"section": "Equity",
"subSection": "Equity",
"account": "Owners’ Equity — Invested Capital",
"value": 1000000
},
{
"section": "Equity",
"subSection": "Equity",
"group": "Net Income",
"account": "Profit for the year",
"value": 37324.72
}
]
}
]
}
API Response Structure
Accounting Data as a Service™ returns all synchronized data in the form of a report structure. Each report contains a
meta
object summarizing the content. See Reports for more details.
Pushing Data
Accounting Data as a Service™ supports creating records in a business' accounting service provider through a set of POST API endpoints. All push endpoints have the following format: POST /{dataType}
(e.g. POST /invoices
)
Anytime you want to push data to a business's accounting service provider, you will need to use the businessName
and serviceName
associated with that business connection to make a request to our API.
As an example, we'll look at posting a new invoice to the business connected in the previous step using the POST /invoices endpoint.
{
"connection":
{
"businessName": "Test Business",
"serviceName": "quickbooks"
},
"data":
{
"invoiceNumber": "INV-200",
"customerRef": "583",
"postedDate": "2021-09-05",
"dueDate": "2021-10-05",
"currency": "CAD",
"lines":
[
{
"description": "Professional services - 2 hrs.",
"unitAmount": 100.5,
"quantity": 2,
"subTotal": 201.5,
"taxAmount": 20.5,
"totalAmount": 222,
"discountPercentage": 10,
"accountRef": "200",
"taxRef": "GST"
}
],
"memo": "Friendly invoice description to customer."
}
}
All push endpoints are implemented to work asynchronously so you will receive a pending push status in response to your push request.
{
"businessName": "Test Business",
"serviceName": "quickbooks",
"pushCommunicationId": "60473b57f0cdd1683ca71f60",
"requestedOn": "2021-03-09T08:15:22.035Z",
"status": "pending",
"data":
{
"invoiceNumber": "INV-200",
"customerRef": "583",
"postedDate": "2021-09-05",
"dueDate": "2021-10-05",
"currency": "CAD",
"lines":
[
{
"description": "Professional services - 2 hrs.",
"unitAmount": 100.5,
"quantity": 2,
"subTotal": 201.5,
"taxAmount": 20.5,
"totalAmount": 222,
"discountPercentage": 10,
"accountRef": "200",
"taxRef": "GST"
}
],
"memo": "Friendly invoice description to customer."
}
}
Managing Data in Accounting Data as a Service™
To learn more about managing data in Accounting Data as a Service™ see before proceeding:
Updated 4 months ago
Congratulations, you have completed the Quickstart! From here, we invite you to learn more about all the features and next steps to get you ready for production.